Vue3+TS+Element-plus
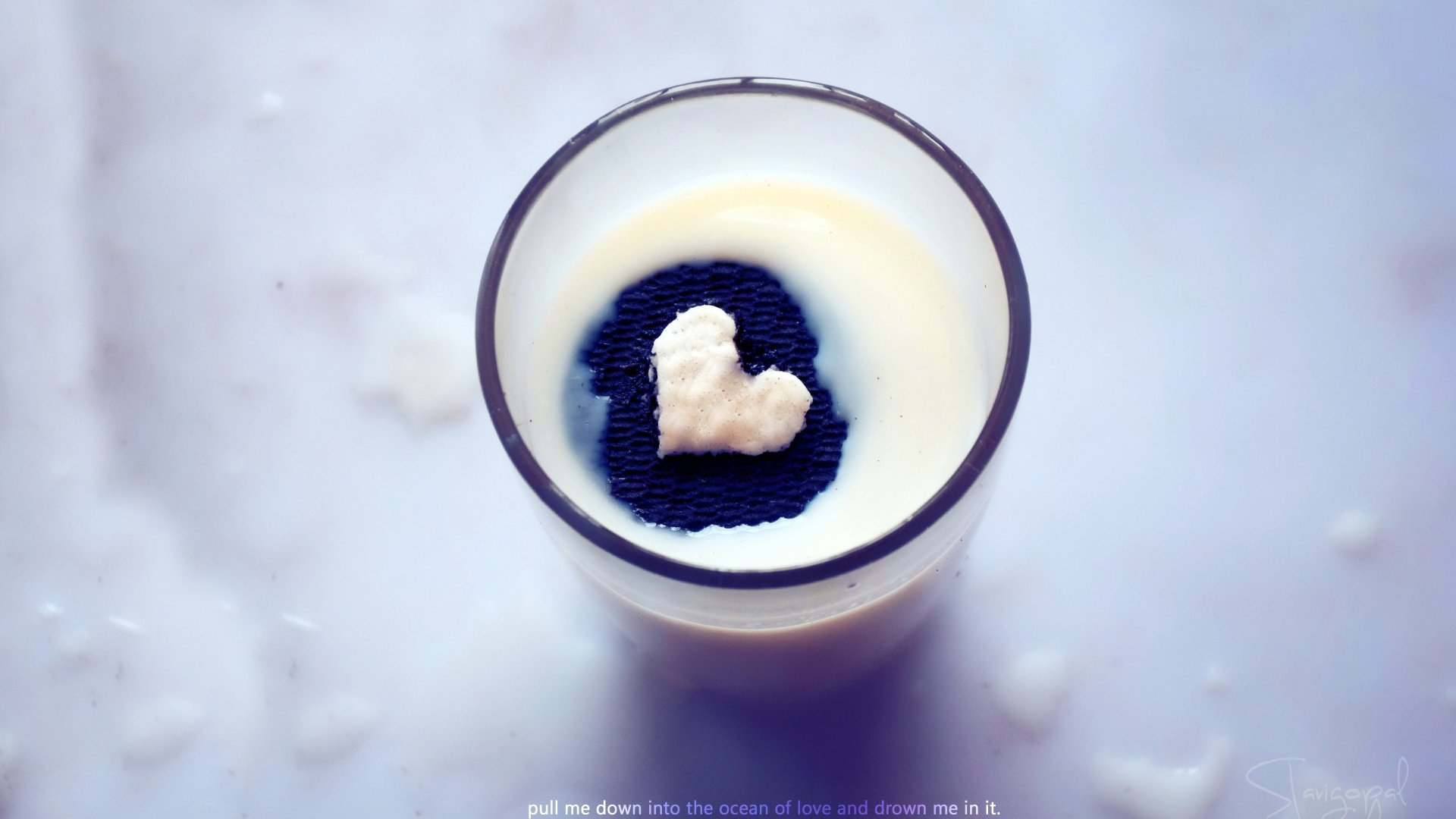
Vue3+TS+Element-plus
Jie一. 设计原则
1. 一致 Consistency
与现实生活一致: 与现实生活的流程、逻辑保持一致,遵循用户习惯的语言和概念;
在界面中一致: 所有的元素和结构需保持一致,比如:设计样式、图标和文本、元素的位置等。
2. 反馈 Feedback
控制反馈: 通过界面样式和交互动效让用户可以清晰的感知自己的操作;
页面反馈: 操作后,通过页面元素的变化清晰地展现当前状态。
3. 效率 Efficiency
简化流程: 设计简洁直观的操作流程;
清晰明确: 语言表达清晰且表意明确,让用户快速理解进而作出决策;
帮助用户识别: 界面简单直白,让用户快速识别而非回忆,减少用户记忆负担。
4. 可控 Controllability
用户决策: 根据场景可给予用户操作建议或安全提示,但不能代替用户进行决策;
结果可控: 用户可以自由的进行操作,包括撤销、回退和终止当前操作等。
二. 导航
导航可以解决用户在访问页面时:在哪里,去哪里,怎样去的问题。 一般导航会有「侧栏导航」和「顶部导航」2 种类型。
1. 选择合适的导航
选择合适的导航可以让用户在产品的使用过程中非常流畅,相反若是不合适就会引起用户操作不适(方向不明确)。 以下是「侧栏导航」和 「顶部导航」的区别。
2. 侧栏导航
可将导航栏固定在左侧,提高导航可见性,方便页面之间切换; 顶部可放置常用工具,如搜索条、帮助按钮、通知按钮等。 适用于中后台的管理型、工具型网站。
a. 一级类目
适用于结构简单的网站:只有一级页面时。 不需要使用面包屑。
b. 二级类目
侧栏中最多可显示两级导航; 侧栏中最多可显示两级导航; 当使用二级导航时,我们建议搭配使用面包屑,方便用户定位自己的位置和快速返回。
c. 三级类目
适用于较复杂的工具型后台。 适用于较复杂的工具型后台,左侧栏为一级导航,中间栏可显示其对应的二级导航,也可放置其他的工具型选项。
3. 顶部导航
顺应了从上至下的正常浏览顺序,方便浏览信息; 顶部宽度限制了导航的数量和文本长度。
适用于导航较少,页面篇幅较长的网站。
三. 安装
1. 兼容性
Element Plus 支持最近两个版本的浏览器。
如果您需要支持旧版本的浏览器,请自行添加 Babel 和相应的 Polyfill 。
由于 Vue 3 不再支持 IE11,Element Plus 也不再支持 IE 浏览器。
2. Sass
在 2.8.5 及以后的版本, Sass 的最低支持版本为 1.79.0.
如果您的终端提示 legacy JS API Deprecation Warning, 您可以配置以下代码在 vite.config.ts 中.
1 | css: { |
3. 使用包管理器
我们建议您使用包管理器(如 NPM、Yarn 或 pnpm)安装 Element Plus,然后您就可以使用打包工具,例如 Vite 或 webpack。
1 | # 选择一个你喜欢的包管理器 |
浏览器直接引入
直接通过浏览器的 HTML 标签导入 Element Plus,然后就可以使用全局变量 ElementPlus 了。
根据不同的 CDN 提供商有不同的引入方式, 我们在这里以 unpkg 和 jsDelivr 举例。 你也可以使用其它的 CDN 供应商
unpkg
1 | <head> |
jsDelivr
1 | <head> |
注意:
由于原生的 HTML 解析行为的限制,单个闭合标签可能会导致一些例外情况,所以请使用双封闭标签,例如:
1 | <!-- examples --> |
四. 快速开始
1. 用法
a. 完整引入
如果你对打包后的文件大小不是很在乎,那么使用完整导入会更方便。
1 | // main.ts |
Volar 支持
如果您使用 Volar,请在 tsconfig.json 中通过 compilerOptions.type 指定全局组件类型。
1 | // tsconfig.json |
b. 按需导入
您需要使用额外的插件来导入要使用的组件。
自动导入(推荐)
推荐
首先你需要安装 unplugin-vue-components 和 unplugin-auto-import 这两款插件
1 | cnpm install -D unplugin-vue-components unplugin-auto-import |
然后把下列代码插入到你的 Vite 或 Webpack 的配置文件中
Vite
1 | // vite.config.ts |
c. 手动导入
Element Plus 提供了基于 ES Module 的开箱即用的 Tree Shaking 功能。
但你需要安装 unplugin-element-plus 来导入样式。 配置文档参考 docs.
1 | App.vue |
1 | // vite.config.ts |
2. 快捷搭建项目模板
https://github.com/element-plus/element-plus-vite-starter
3. 全局配置
在引入 ElementPlus 时,可以传入一个包含 size
和 zIndex
属性的全局配置对象。 size
用于设置表单组件的默认尺寸,zIndex
用于设置弹出组件的层级,zIndex
的默认值为 2000
。
a. 完整引入:
1 | import { createApp } from "vue"; |
b. 按需引入:
1 | <template> |